Eigentümer einer Maildatei können Zugriffe auf Mails und Kalender über “Werkzeuge – Vorgaben – Zugriff und Delegierung” selbstständig steuern.
Bei der geplanten Archivierung von Mails ergeben sich daraus aber Probleme beim Zugriff auf archivierte Dokumente.
Ein Archivsystem sichert neben den eigentlichen Inhalten der Mail auch die zu diesem Zeitpunkt existierende ACL.
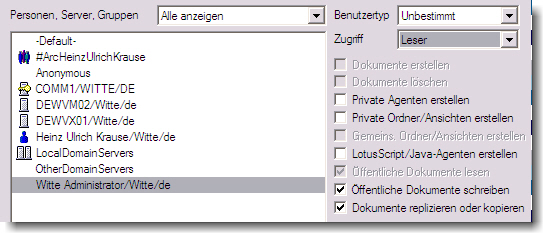
Lt. der abgebildeten ACL darf also neben dem Eigentümer der Maildate auch der Administrator lesend auf die Mails zugreifen und somit auch auf archivierte Mails.
Wird der Zugriff für den Leser Administrator wieder entfernt, so kann er weder auf die Datenbank noch auf Mails zugreifen, die nach diesem Zeitpunkt archiviert wurden. Sehr wohl kann er aber noch über die Archivrecherche auf Mails zugreifen, die vor dem Zeitpunkt der Löschung seines Zugriffs aus der ACL in das Archiv gespeichert wurden.
Die Probleme, die sich daraus ergeben können, sollen hier nicht weiter erläutert werden.
Ein in der Praxis wohl häufiger auftretendes Problem erfordert aber unbedingte AUfmerksamkeit; eine Lösung muss VOR Beginn der Mailarchivierung implementiert werden.
Dabei geht es um folgendes Szenario:
Ein Vorgesetzter delegiert den Zugriff auf seine Mails an eine Assistentin, die namentlich in die ACL eingetragen wird. Ã?ber einen gewissen Zeitraum werden nun Mails mit diesen Zugriffsberechtigungen archiviert.
Die bisherige Assistentin wird dann einem neuen Vorgesetzten zur Seite gestellt, oder vertritt eine Kollegin.
In Variante 1 hat dann die neue Assistentin keinen Zugriff auf die bisher archivierten Mails, weil sie bisher ja keinen Zugriff auf die Datenbank ihres Vorgesetzen hatte.
In Variante 2 kann nicht auf die archivierten Mails der zusätzlichen Datenbank zugegriffen werden, weil es an den nötigen Berechtigungen zum Zeitpunk der Archivierung fehlt.
Lösen lässt sich dieses Dilemma, wenn man in die ACL der Datenbank eine Gruppe einfügt, die auf die Datenbank Lesezugriff hat.
Hier stösst man aber zugleich auf ein weiteres Problem. Alle Einträge, die in der ACL nicht als MANAGER oder ENTWICKLER eingetragen sind, können vom Eigentümer der Datenbank über die Vorgaben geändert werden. Das betrifft sowohl die Art des Zugriffs als auch das Löschen des Eintrags.
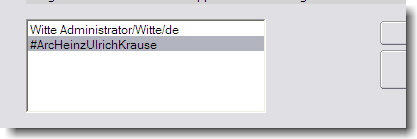
Da wir aber der Gruppe keine Berechtigung als Manager geben wollen, müssen wir anderweitig sicherstellen, daÃ? der MailBox Eigentümer die Rechte der Gruppe nicht ändern kann.
Das kann am Einfachsten dadurch erreicht werden, daÃ? die Gruppe für den Eigentümer gar nicht sichtbar ist.
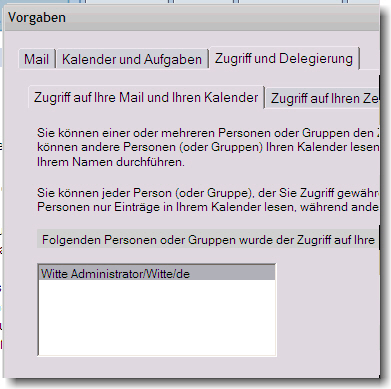
Mit einem kleinen Eingriff in die Gestaltung der Maildatei kommt man zum Ziel. Bei Einführung einer Mailarchivierung wird man in der Regel sowieso die Maildatei modifizieren müssen; bei der einen Lösung mehr, bei einer anderen weniger.
Die Modifikation zum Verstecken der Gruppe(n) sind so minimal, daÃ? sie im Prinzip nicht ins Gewicht fallen. Trotzdem sollten die Codeänderungen dokumentiert werden. 🙂
In der Script Bibliothek “ACLManagement” wird der Code ab Zeile 578 ( +/- 2 Zeilen ) folgendermaÃ?en geändert
alt:
Case ACLLEVEL_READER
x = Ubound(Me.ReadMail)
Me.ReadMail(x) = Me.aclentry.Name
Redim Preserve Me.ReadMail(x+1)
Call IsPublicReader()
Call IsPublicWriter()
neu:
Case ACLLEVEL_READER
x = Ubound(Me.ReadMail)
'############## 26.10.2007,Ulrich Krause ################
If ( Not Instr(Me.aclentry.Name, "#Arc" ) > 0 ) Then
Me.ReadMail(x) = Me.aclentry.Name
Redim Preserve Me.ReadMail(x+1)
Call IsPublicReader()
Call IsPublicWriter()
End If
Durch diese einfache Ã?nderung ist es nun möglich, in die ACL der Maildatenbanken Gruppen einzufügen, die mit dem Prefix “#Arc” beginnen. Der Eintrag in das Domino Directory und die Pflege der Gruppenmitglieder erfolgt dann bei Bedarf manuell.
Der Name der Gruppe wird aus dem Prefix und z.B. den Namen des Mail Box Eigentümers gebildet. Damit lässt sich dann der Zugriff datenbankspeziefisch steuern. Eine weitere Gruppe “#ArcDefaultReaders” mit ACLLEVEL_READER kann dann in gleicher Weise zur datenbankübergreifenden Recherche verwendet werden.
Dies ist die aus meiner Sicht einfachste Lösung um sicherzustellen, daÃ? unterschiedliche Personen auf archivierte Mails zugreifen können.